- Published on
- View count
- 4469 views
Use a Lite YouTube Embedded Player in Next.js
- Authors
- Name
- Francisco Moretti
- @franmoretti_
Using a Youtube Embedded Player in a Next.js application
I needed a way to play videos locally in a page where I post notes I take from conference talks.
The screenshot shows a video player at the top of a post page.
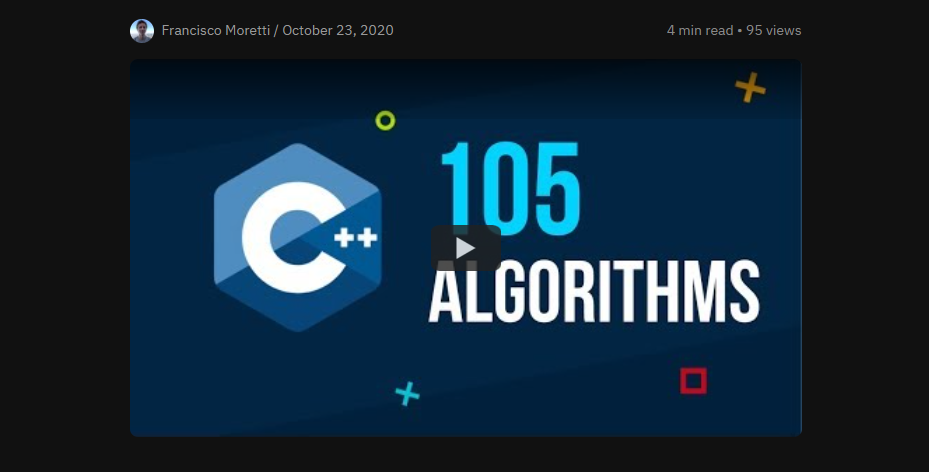
Method 1: IFrame Player
The first approach I took was to use the <iframe>
HTML tag as suggested by YouTube’s developer guide.
The code to use that first method is something like this.
<iframe
src={"https://www.youtube.com/embed/" + video_id}
title={video_title}
frameBorder="0"
allow="accelerometer; autoplay; clipboard-write; encrypted-media; gyroscope; picture-in-picture"
allowFullScreen
></iframe>
You should find the video_id
of the video you want to embed. The video_title
can be anything you want, but I used the title from the video. There are many other options like linking playlists. If you want to learn more about it, check the Supported Parameters reference.
If you want the component to behave responsively, you should fix its aspect ratio. Becaise my application was using tailwindcss, an elegant solution I’ve found for it was to use the tailwindcss-aspect-ratio plugin. You can wrap the IFrame in a div like this one.
<div className=" w-full aspect-w-16 aspect-h-9">
iframe
Performance with The main disadvantage from this method is that it caused a decrease of performance. A Lighthouse report showed the following problem. > some third-party resources can be lazy loaded with a facade
I also saw a small delay to load the player when I opened the webpage in my PC.
Here is an image from the report with more details from the issue.
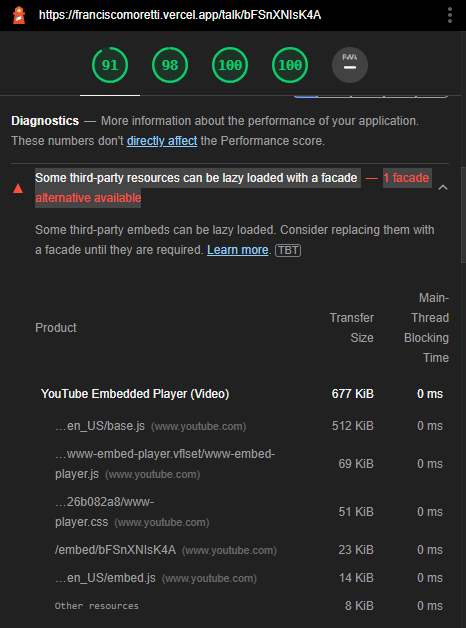
This method is still useful if you don’t mind the small degradation in performance. Also, there are many ways to lazy load it which can solve this issue.
react-lite-youtube-embed
package
Method 2: The After trying a few alternatives I’ve found the react-lite-youtube-embed package which was everything I needed.
This package is a React component created from lite-youTube-embed, a more popular package that has a general web solution to the problem.
This package is really easy to use.
Firstly, you need to install it
yarn add react-lite-youtube-embed
or
npm install react-lite-youtube-embed -S
Secondly, import the packages
import LiteYouTubeEmbed from "react-lite-youtube-embed"
import "react-lite-youtube-embed/dist/LiteYouTubeEmbed.css"
Finally, use the LiteYouTubeEmbed
component. This component also has the option to set the aspect ratio in it, which is extremely useful.
<LiteYouTubeEmbed
aspectHeight="{9}"
aspectWidth="{16}"
id="{video_id}"
title="{video_title}"
/>
It also has some privacy settings enabled by default, it will try to reach youtube-nocookie.com
. Those might conflict with your Next.js config ContentSecurityPolicy. You might need to add it the permission to reach that page to the rules.
react-lite-youtube-embed
Performance with The Lighthouse report with the LiteYouTubeEmbed
component had a sweet 7% increase in performance.
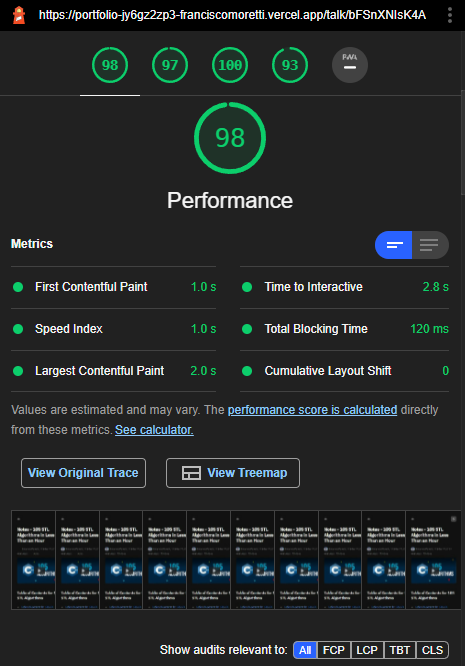
Conclusion
Both methods are great and simple to use. The performance is comparable for both methods. The loading speed is shorter for the second one, but you need to add a package to your project.